AutoAPI
AutoAPI is an innovative tool that facilitates communication between database tables and CUBE models (KUBES) on the client side. It enables efficient and customized display and presentation of information on the screen through automatic communication between tables and cube models. This means you don't need to perform complex queries or manual data manipulations.
How to use
Step 1 Go to the screen to create the AutoAPI.
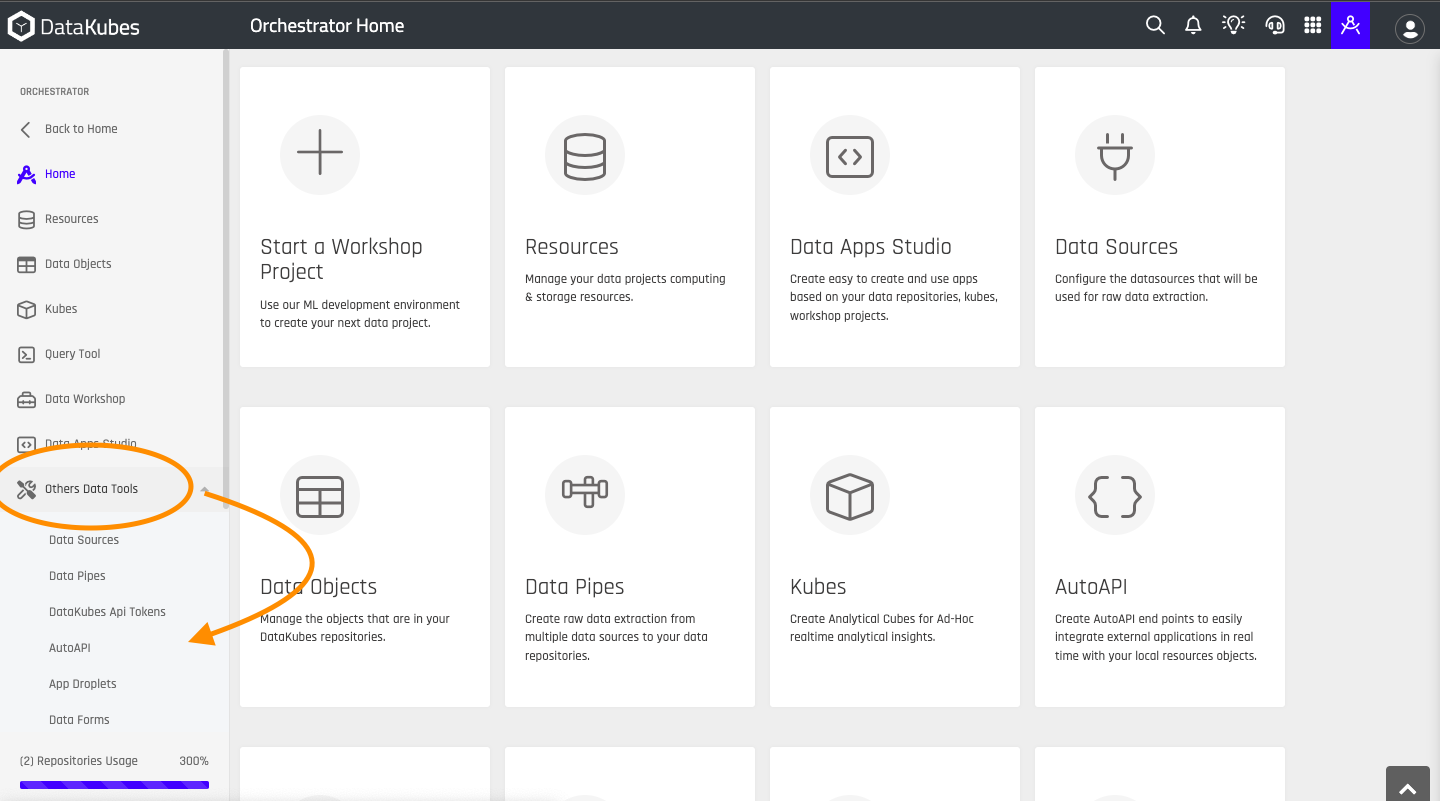
Step 2 Create the AutoAPI.
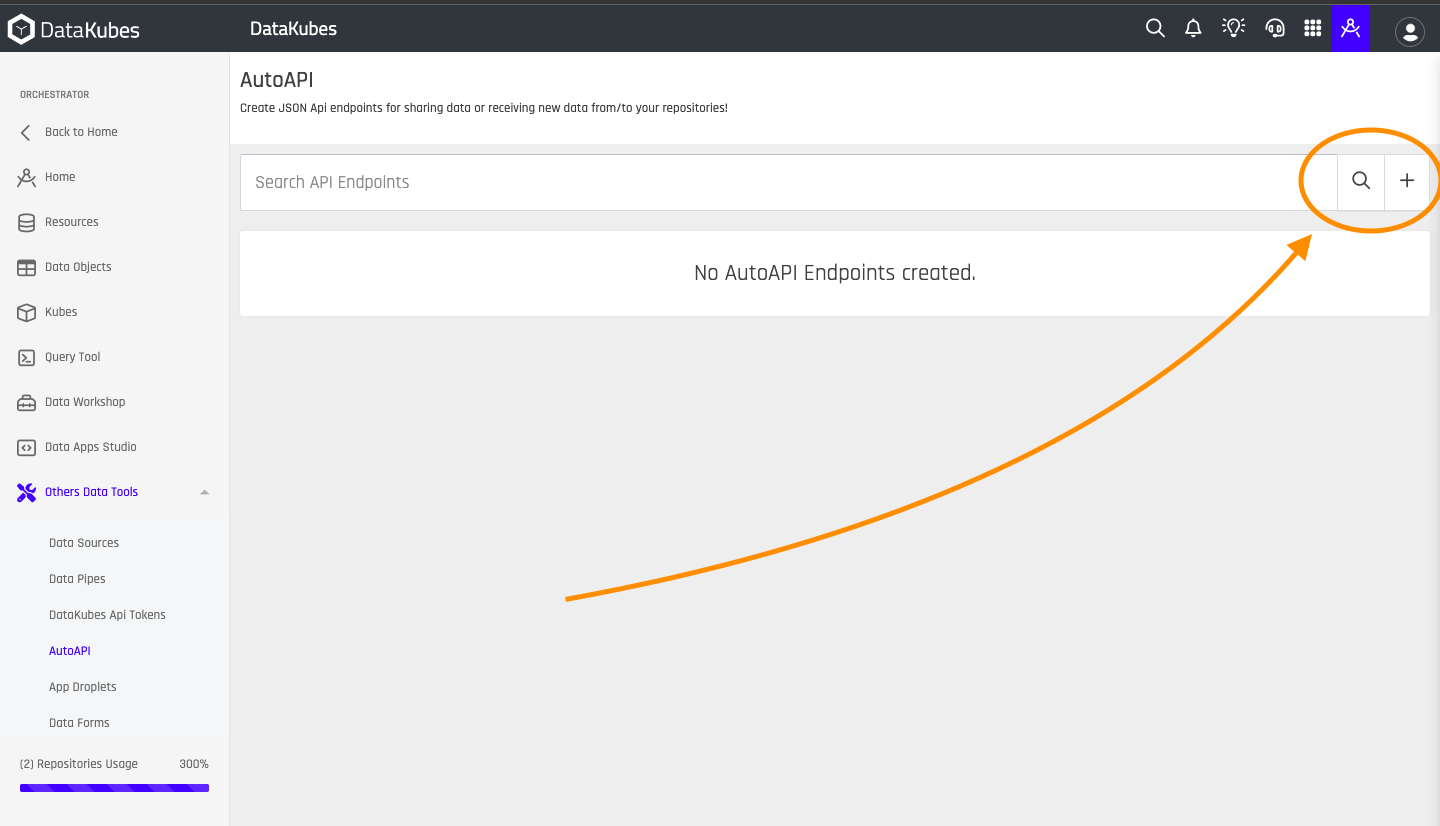
Step 3 Add the necessary information for your AutoAPI and select a method (Endpoint)
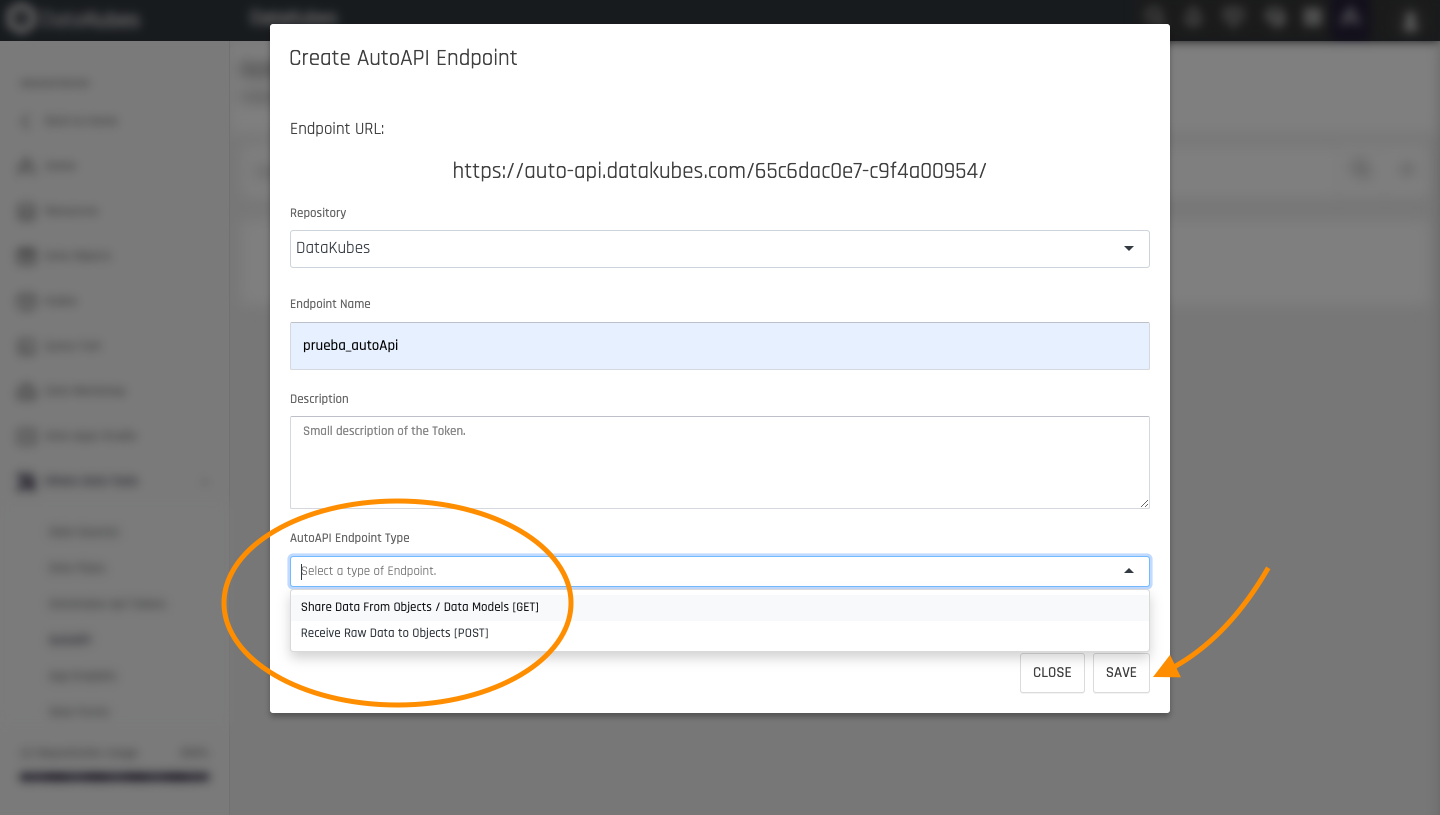
Step 4 Select your autoAPI to access the settings
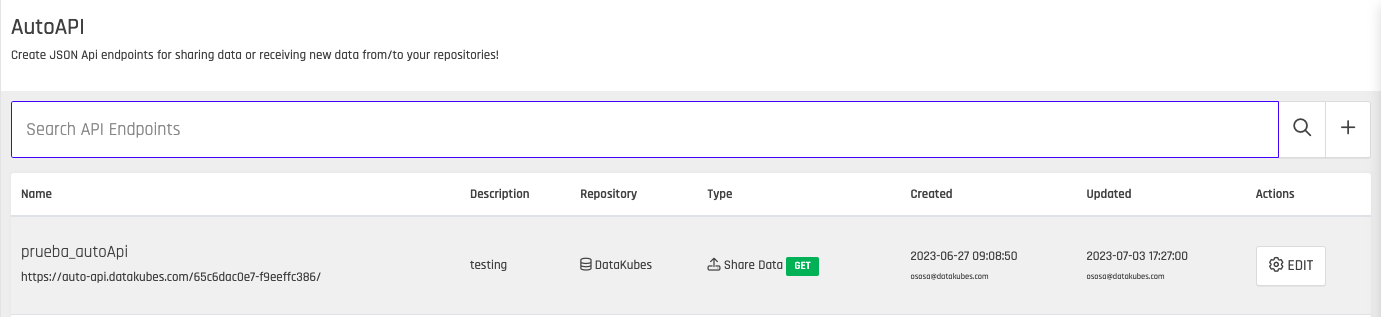
Step 5 Enter configuration in the case of making an autoAPI of type GET
- Select between one or more tables or a cube (KUBE) to provide and update the information
- Select your columns to share in your autoAPI
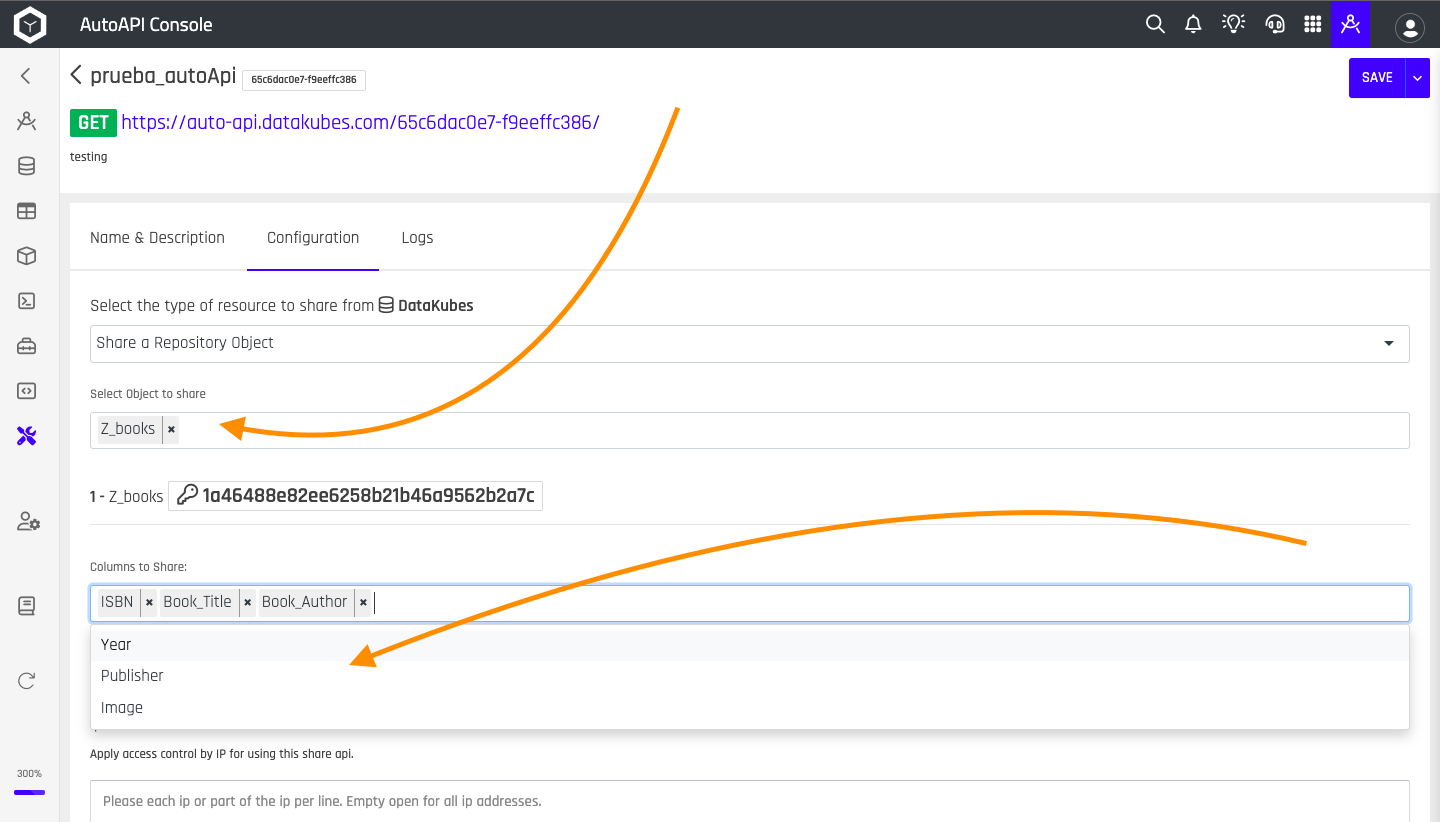
Step 6 Save the changes to be able to use your autoAPI!
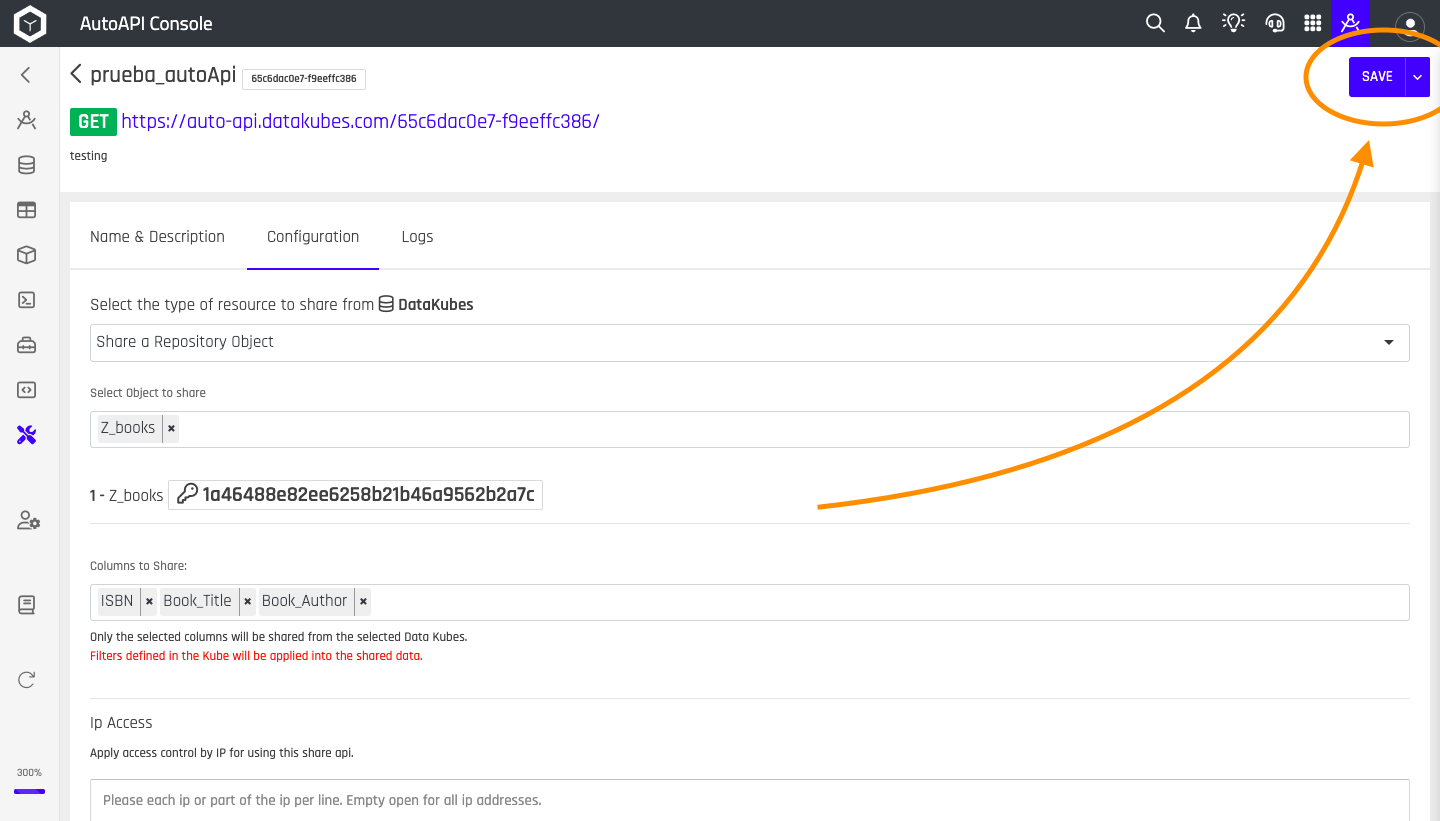
Enter configuration in the case of making an autoAPI of type POST these are the steps to follow:
- Once the first load of type POST is done, the events will be enabled
- These events are responsible for modeling the information according to your request POST
- After selecting your event you can use between two upload models (Single / Multiple Rows) depending on the User's need, the (Single) can upload data individually and the (Multiple Rows) can upload multiple data in a massive way in an optimized way thanks to the AutoAPI
- Then select which property will be added to the columns of your table
- Save your changes.
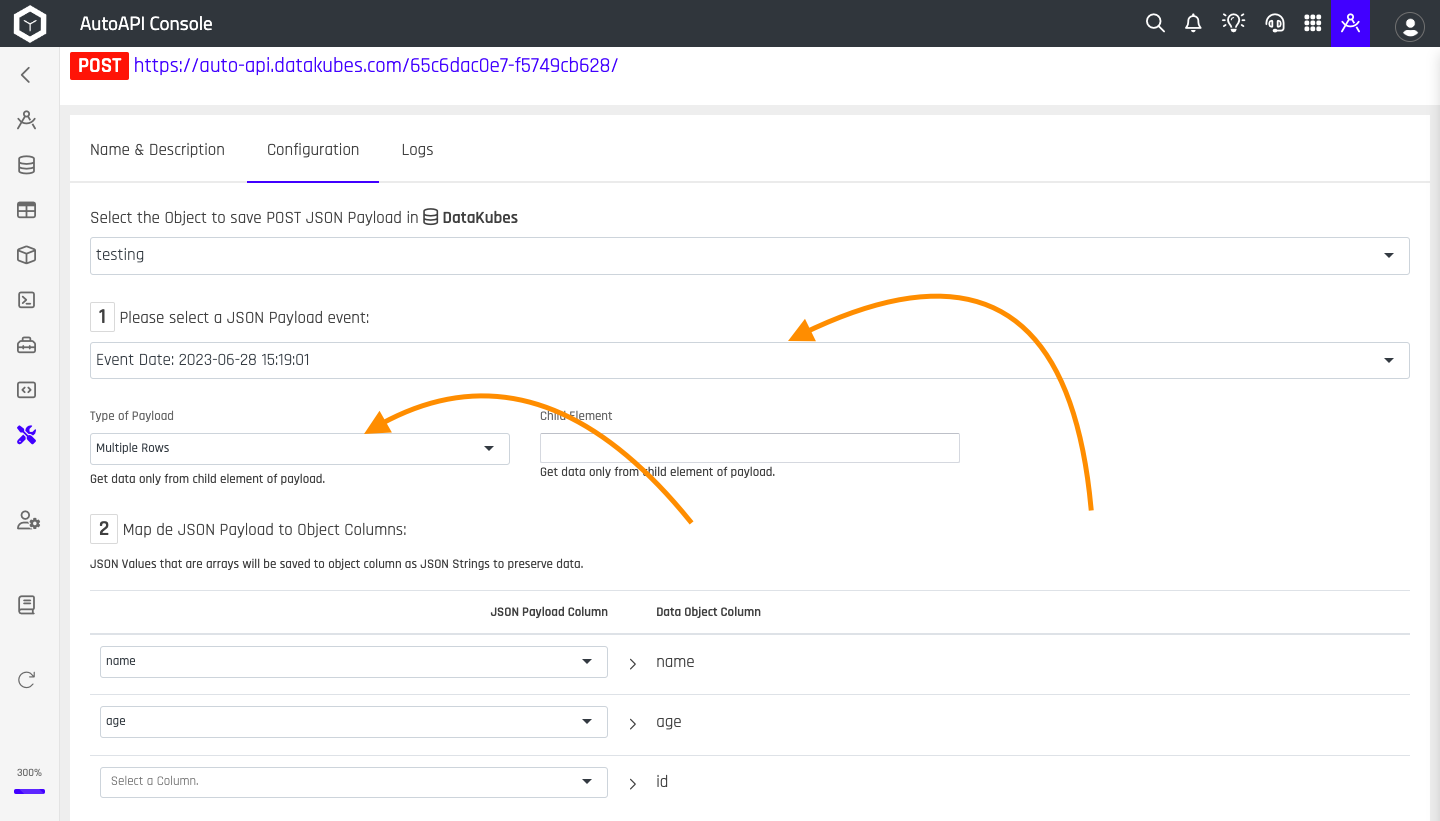
Endpoint
GET It is used to retrieve data from a table or cube (Kube). When you make a GET request, you are asking the server to return a specific resource or information, either individually or in bulk.
GET URL
The URL is divided into three parts:
BASE: It is the main part of the URL that indicates the base address of the server to which the request will be made.
<https://auto-api.datakubes.com>
TOKEN: It is a parameter in the URL that represents a unique access token and is used to authenticate and authorize the request.
?token=65c6dac0e7-f9eeffc386
SHARED TOKEN: It is another parameter in the URL that represents a shared token, which can be used to share access or perform certain specific actions.
&shared_token=1a46488e82eaddio219430742e6246a9562b2a7c
AUTOAPI: unified url and parameters:
<https://auto-api.datakubes.com?token=65c6dac0e7-f9eeffc386&shared_token=1a46488e82eaddio219430742e6246a9562b2a7c>
GET URL Queries
Field | Description | Default Value | Required |
---|---|---|---|
Token | Authorization token created from Security / Api Tokens. | X | |
Shared_token | Token of the Kube resource either Reports or Kube Store Tables. | X | |
results | Number of records to get | Empty equals 100. | |
groupby | groups by one or more fields in columns | Empty without grouping | |
page | Page that you want to obtain according to the requested results, for example in a results=100 page=2 it will show from 100 to record 200 | Empty equals 1. | |
filters | JSON array with the filters to be applied in the case of the Payload model or URL GET variable array in the case of direct integration | Empty without filters. | |
orderby | JSON array with field object to sort in ascending and descending order, for example\
| Empty without ordering and only valid fields and type ordering are accepted |
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://auto-api.datakubes.com/<Your Api Token >',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_POSTFIELDS =>'{
"results": 100,
"groupby": {
"cols": [
"IdProduct",
"ProductName"
]
},
"orderby": [
{
"field": "ProductName",
"type": "ASC"
}
],
"filters": [
{
"field": "ClientId",
"type": "=",
"value": "C0088"
}
],
"report_type": "groupby"
}',
CURLOPT_HTTPHEADER => array(
'Authorization: Bearer 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',
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
$.get('https://auto-api.datakubes.com/<Your Api Token >', {
results: 100,
groupby: {
cols: ['IdProduct', 'ProductName']
},
orderby: [
{
field: 'ProductName',
type: 'ASC'
}
],
filters: [
{
field: 'ClientId',
type: '=',
value: 'C0088'
}
],
report_type: 'groupby'
})
.done(function(response) {
console.log(response);
})
.fail(function(xhr, status, error) {
console.log('Error:', error);
});
{
/* server response */
"result": "OK",
"data": [
{
"ClientName": "User 1",
"ClientId": "C0548",
"ProductId": "PS0000020",
"ProductName": "NESPRESSO CATUAI 10 CAPSULAS ",
"Item_Number": "8055732940904",
"Count": "1"
},
{
"ClientName": "User 1",
"ClientId": "C0548",
"ProductId": "PS0000021",
"ProductName": "COFFEE HAT MOLIDO 250G 100% jmj",
"Item_Number": "8050508250349",
"Count": "1"
},
{
"ClientName": "User 1",
"ClientId": "C0548",
"ProductId": "PS0000109",
"ProductName": "CONFETTI ALMENDRA FONDENTE 285 GRAMOS",
"Item_Number": "8050538250721",
"Count": "1"
}
],
/* objeto de paginación */
"total_rows": {
"count": "16266",
"pages": 163
}
}
POST
It is used to send data to the server to create a new resource or perform a specific action on the database. When you make a POST request, you are sending data in the request body for the server to process.
$data = array(
array("name" => "Example 1", "age" => 25),
array("name" => "Example 2", "age" => 30),
array("name" => "Example 3", "age" => 35)
);
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://auto-api.datakubes.com/65c6dac0e7-f5749cb628/",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => json_encode($data),
CURLOPT_HTTPHEADER => array(
"Content-Type: application/json"
)
));
$response = curl_exec($curl);
$error = curl_error($curl);
curl_close($curl);
if ($error) {
echo "Request error: " . $error;
} else {
echo "Server response: " . $response;
}
/* solicitud POST */
var data = [
{ "nombre": "Example 1", "age": 25 },
{ "nombre": "Example 2", "age": 30 },
{ "nombre": "Example 3", "age": 35 }
];
$.post("https://auto-api.datakubes.com/65c6dac0e7-f5749cb628/",JSON.stringify(data), function(response) {
console.log("Server response:", response);
}).fail(function(error) {
console.error("Request error:", error);
});
{
/* server response */
"result": "OK",
}
Details to be considered:
Field Name Errors
The fields must be sent the way they are created in the table in the repository. In the event that a field is not named correctly or does not exist, it will send an error in the API.
Primary Keys
In the event that the destination table in the repository has a primary key and there is data sent in the API, it will replace the data of said unique record.
Records with incomplete fields!
In the event that you send a data record where not all the fields or columns are contained in the payload sent to the API, they will be filled with predefined, null or empty values depending on how they have been configured in the table.
Array of objects in JSON format
remember to use the JSON format when using the AutoAPI
Updated 4 months ago